Tools¶
Java >= 8¶
This year you will work with the new version of java : Java 8.
To develop with Java 8 you need to download and install the java development kit JDK 12. You just need to agree the license and download the version that correspond to your distribution:
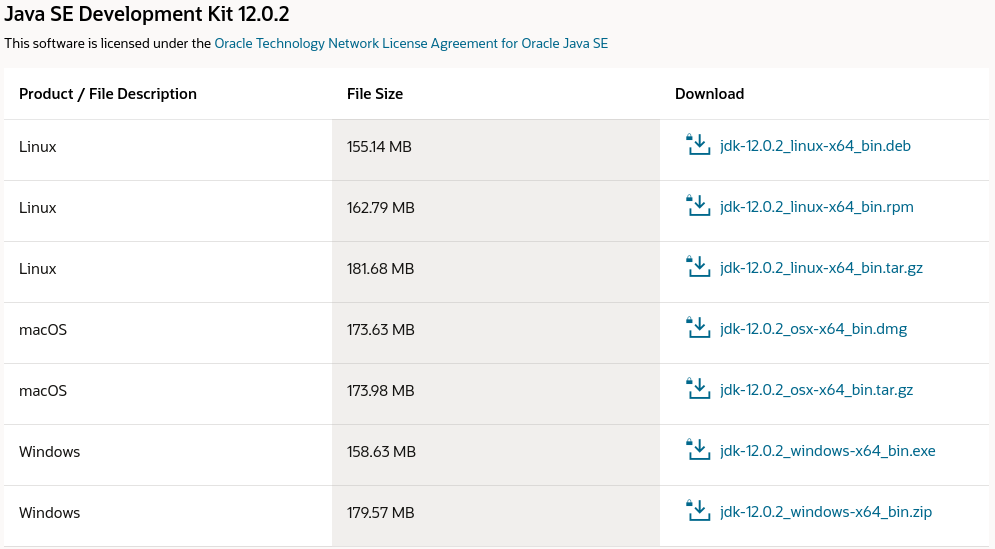
After downloading it, just follow the instructions of the installation.
IDE IntelliJ¶
You can develop java with any text editor but we strongly recommend you to use an IDE and more specificly IntelliJ. That’s the only supported editor at the exam. To use all functionalities of this one, you can get a free student license with your uclouvain student mail.
Download and install IntelliJ then follow this video that explain you how to start with IntelliJ. Try to create a new project and run a Hello world program using the arguments passed to the program like in the video.
Debugging¶
Strategy¶
Take a simple problem that you know the result.
For example take the follow code that calculates the maximum element of an array of integers. This code looks like great on the first look but inginious says that your function isn’t correct … Why?
private static int max(int[] array){ int max = 0; for(int i : array){ max = Math.max(max, i); } return max; }
Let’s take a first example and try to run it :
public static void main(String... args){ int[] a1 = {5, 6, 20, 1, 40}; System.out.println(max(a1)); //prints 40 }
It seems good … let’s take another example, maybe with some negative integers :
public static void main(String... args){ int[] a2 = {5, 6, -20, 1, -40}; System.out.println(max(a1)); //prints 6 }
Another good answer … what if we take an entirely negative array :
public static void main(String... args){ int[] a1 = {-5, -6, -20, -1, -40}; System.out.println(max(a1)); //prints 0 }
Damn, that’s not what we want. The good answer here should be -1. Now take a new look to our max function. We can see that the local variable max has the value 0 to start. So when the array is composed of only negative integers, the return value is always 0, and that’s not what we want. So let’s correct this :
private static int max(int[] array){ int max = Integer.MIN_VALUE; for(int i : array){ max = Math.max(max, i); } return max; } public static void main(String... args){ int[] a1 = {-5, -6, -20, -1, -40}; System.out.println(max(a1)); //prints -1 }
You can see that here, to debug our program, we use simple examples that we know the solution to test our code. On small problems like this one it’s an easy and fast solution to debug but on bigger programs, it’s not a maintainable solution.
Use Tests.
For bigger programs a good practice is to create tests that covers the whole execution of the program. In java the most used library is Junit 5. To create and run tests on IntelliJ looks at this tutorial.
We can take as example the previous function max to show you an example of test.
import org.junit.jupiter.api.Assertions; import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; class DebuggingTest { @Test public void testMax(){ int[] positiveArray = {5, 6, 20, 1, 40}; Assertions.assertEquals(Debugging.max(positiveArray), 40); int[] positiveAndNegativeArray = {5, 6, -20, 1, -40}; Assertions.assertEquals(Debugging.max(positiveAndNegativeArray), 6); int[] negativeArray = {-5, -6, -20, -1, -40}; Assertions.assertEquals(Debugging.max(negativeArray), -1); } }
Look at the Junit 5 for more examples and details about JUnit.
Use Assert inside your code.
Thanks to JUnit, it’s also possible to verify invariant of your programs. For example we know that at each iteration of the loop inside max, \(max >= array[i]\). We can verify this invariant inside the loop (we have modify the above example, try to find why the invariant is not respected here) :
public static int max(int[] array){ int max =0; for(int i = 0; i<array.length; i++){ if(max > array[i]){ max = array[i]; } assertTrue(max > array[i]); } return max; }
Use breakpoints.
A last way to debug your programs is to use breakpoints inside IntelliJ. The breakpoints allow you to halt the execution of your program at a location that you define to check the state of the execution. You can find here a complete tutorial on how to use breakpoints in IntelliJ.
Avoid problems¶
Work with a structure.
When you start programming, the simplest mistake you do is going on your computer and start writing lines of code. It is common to see a beginner taking his computer and try to write lines before finishing to read the instructions.
If you want to be efficient when you try to solve a problem, you should start by thinking about the problem. Take a sheet of paper, write pseudo-code, think about examples of the problem given to you. How would you solve it? What are the difficulties? What should you not forget?
Finally when you start having a good idea of the problem and how you want to solve it, you can start to consider taking your computer!
Make your code readable and write comments.
You work on an Inginious exercise and you have to stop because your room-mate wants to go to the beer bar to test the new drafted beer. You follow him and a few days later, you restart working on the exercise but your code looks like this:
public static int doSomething(int[] x){ int anotherValue = x.length; int someValue = Integer.MIN_VALUE; for(int i = 0; i< anotherValue; i++){ someValue = someValue<= x[i] ? x[i] : someValue ; } return someValue; }
Well, not easy to read? And now you have to take a lot of time to understand the code you’ve written! It is even harder to find errors in a code like this.
What are the good practices in terms of programming that helps you to make your code readable and also easy to correct?
First you should choose the name of your methods and variable wisely, in the example we give naming the method “maxFinder” or “maximum” is a good way to know what this method should do. For the variable we can choose “len” for the array length, “max” instead of “someValue” and “array” instead of “x” for the array name. Here is how your code is going to look:
public static int maxFinder(int[] array){ int len = array.length; int max = Integer.MIN_VALUE; for(int i = 0; i< len; i++){ max = max<= array[i] ? array[i] : max ; } return max; }
It already looks way easier to read but we can make it even more readable by writing comments:
public static int maxFinder(int[] array){ int len = array.length; int max = Integer.MIN_VALUE; //Initializing max to the minimum integer value 2^(31)-1 for(int i = 0; i< len; i++){ //Iterating through array max = max<= array[i] ? array[i] : max ; //Replacing max if we have a bigger value } return max; }
Now you have a code that is easy to read and understand by you or by someone else. When you work on a group project it is very important to be able to read someone else’s code, but if the code looks like the first example it makes you way less efficient.
You should read this article about the good practices when coding in Java.
Maven and Gradle¶
Maven and Gradle are two build automation tools for java projects. They describe how software are built and their dependencies. You probably will not have to use these tools in this course but if you want to take a look on how to configure a project based on these tools, watch these videos : Working with maven in IntelliJ, Working with maven in IntelliJ.
Gradle is among other used for android development.